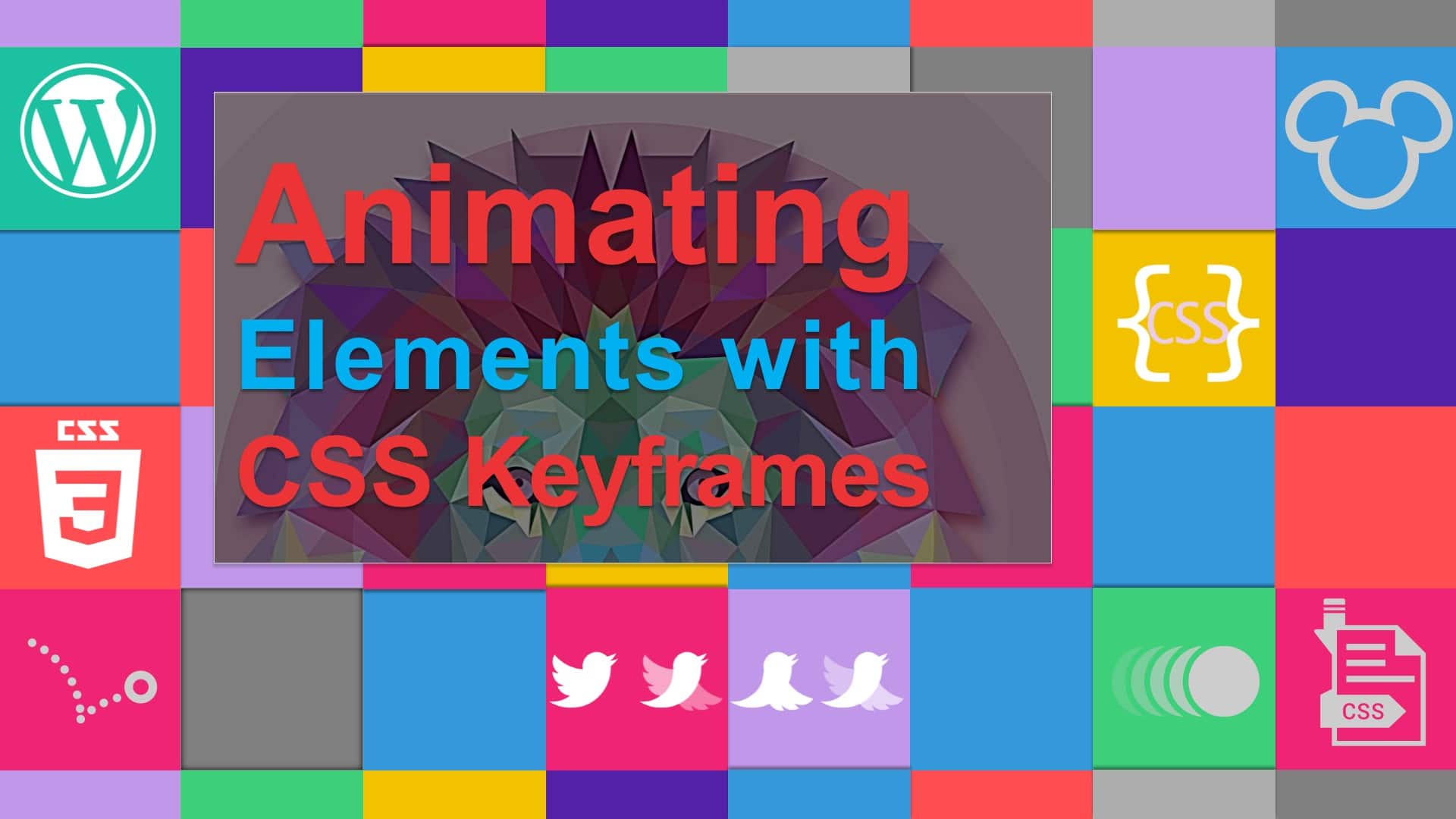
Syntax and What it Does.
Animation in CSS helps you manipulate an element on your website in a stylish way. Whether you want an element to bounce up and down ten times, or continuously change colors, Keyframes help you do that.
Syntax
.yourCustomClassOrID{
animation-name: myAnimationName;
animation-duration: 6s ; /* or 6000ms */
animation-iteration-count: 2; /* or infinite */
animation-direction: normal; /* or alternate */
animation-delay: 1s; /* or 1000ms */
animation-fill-mode: both; /* or backwards, forwards, none */
animation-timing-function: ease; /* or ease-in, ease-out, ease-in-out, linear, cubic-bezier()*/}
However, there is a shorthand for this property, and it goes like this:
.yourCustomClassOrID{
animation: myAnimationName duration delay iteration-count direction fill-mode;}
Order doesn’t matter if you don’t have your duration and delay set, otherwise you have to follow this order.
To start styling your animation, we use the @keyframes rule, which is what manipulates the element from one style to another:
@keyframes myAnimationName{
from{ color: white }
to{ color: red }
/*From white color to red color within the duration you set in the animation property */}
Sounds easy right? Well, for this property, you also need to include prefixes to support cross-browser compatibility, and the way you initialize prefixes is the following:
.yourCustomeClassOrID{
-webkit-animation: myAnimationName 3s infinite; /* Safari 4.0 – 8.0 */
-o-animation: myAnimationName 3s infinite; /* Opera 12.0+ */
-moz-animation: myAnimationName 3s infinite; /* FireFox 5.0+ */
animation: myAnimationName 3s infinite; /* IE 10.0+ */}
@-webkit-keyframes yourAnimationName{
0%{ background-color: red }
50%{ background: yellow }
100%{ background: blue }
}
@-moz-keyframes yourAnimationName{
0%{ background-color: red }
50%{ background: yellow }
100%{ background: blue }
}
@-o-keyframes yourAnimationName{
0%{ background-color: red }
50%{ background: yellow }
100%{ background: blue }
}
@keyframes yourAnimationName{
0%{ background-color: red }
50%{ background: yellow }
100%{ background: blue }
}
/*Any % from 0% to 100% can be used to set intervals between each animation*/
Animation Example
This concludes Animating with keyframes. The only limit to animating with keyframes is your imagination, so go out and and start animating!